Java Benchmark 基准测试的实例详解
import java.util.Arrays; import java.util.concurrent.TimeUnit; import org.openjdk.jmh.annotations.Benchmark; import org.openjdk.jmh.annotations.BenchmarkMode; import org.openjdk.jmh.annotations.Measurement; import org.openjdk.jmh.annotations.Mode; import org.openjdk.jmh.annotations.OutputTimeUnit; import org.openjdk.jmh.annotations.Scope; import org.openjdk.jmh.annotations.State; import org.openjdk.jmh.annotations.Threads; import org.openjdk.jmh.annotations.Warmup; import org.openjdk.jmh.runner.Runner; import org.openjdk.jmh.runner.RunnerException; import org.openjdk.jmh.runner.options.Options; import org.openjdk.jmh.runner.options.OptionsBuilder; @BenchmarkMode(Mode.Throughput)//基准测试类型 @OutputTimeUnit(TimeUnit.SECONDS)//基准测试结果的时间类型 @Warmup(iterations = 3)//预热的迭代次数 @Threads(2)//测试线程数量 @State(Scope.Thread)//该状态为每个线程独享 //度量:iterations进行测试的轮次,time每轮进行的时长,timeUnit时长单位,batchSize批次数量 @Measurement(iterations = 2, time = -1, timeUnit = TimeUnit.SECONDS, batchSize = -1) public class InstructionsBenchmark{ static int staticPos = 0; //String src = "SELECT a FROM ab , ee.ff AS f,(SELECT a FROM `schema_bb`.`tbl_bb`,(SELECT a FROM ccc AS c, `dddd`));"; final byte[] srcBytes = {83, 69, 76, 69, 67, 84, 32, 97, 32, 70, 82, 79, 77, 32, 97, 98, 32, 32, 32, 32, 32, 32, 32, 32, 32, 32, 32, 32, 32, 44, 32, 101, 101, 46, 102, 102, 32, 65, 83, 32, 102, 44, 40, 83, 69, 76, 69, 67, 84, 32, 97, 32, 70, 82, 79, 77, 32, 96, 115, 99, 104, 101, 109, 97, 95, 98, 98, 96, 46, 96, 116, 98, 108, 95, 98, 98, 96, 44, 40, 83, 69, 76, 69, 67, 84, 32, 97, 32, 70, 82, 79, 77, 32, 99, 99, 99, 32, 65, 83, 32, 99, 44, 32, 96, 100, 100, 100, 100, 96, 41, 41, 59}; int len = srcBytes.length; byte[] array = new byte[8192]; int memberVariable = 0; //run public static void main(String[] args) throws RunnerException { Options opt = new OptionsBuilder() .include(InstructionsBenchmark.class.getSimpleName()) .forks(1) // 使用之前要安装hsdis //-XX:-TieredCompilation 关闭分层优化 -server //-XX:+LogCompilation 运行之后项目路径会出现按照测试顺序输出hotspot_pid<PID>.log文件,可以使用JITWatch进行分析,可以根据最后运行的结果的顺序按文件时间找到对应的hotspot_pid<PID>.log文件 .jvmArgs("-XX:+UnlockDiagnosticVMOptions", "-XX:+LogCompilation", "-XX:+TraceClassLoading", "-XX:+PrintAssembly") // .addProfiler(CompilerProfiler.class) // report JIT compiler profiling via standard MBeans // .addProfiler(GCProfiler.class) // report GC time // .addProfiler(StackProfiler.class) // report method stack execution profile // .addProfiler(PausesProfiler.class) //.addProfiler(WinPerfAsmProfiler.class) //更多Profiler,请看JMH介绍 //.output("InstructionsBenchmark.log")//输出信息到文件 .build(); new Runner(opt).run(); } //空循环 对照项 @Benchmark public int emptyLoop() { int pos = 0; while (pos < len) { ++pos; } return pos; } @Benchmark public int increment() { int pos = 0; int result = 0; while (pos < len) { ++result; ++pos; } return result; } @Benchmark public int decrement() { int pos = 0; int result = 0; while (pos < len) { --result; ++pos; } return result; } @Benchmark public int ifElse() { int pos = 0; int result = 0; while (pos < len) { if (pos == 10) { ++result; ++pos; } else { ++pos; } } return result; } @Benchmark public int ifElse2() { int pos = 0; int result = 0; while (pos < len) { if (pos == 10) { ++result; ++pos; } else if (pos == 20) { ++result; ++pos; } else { ++pos; } } return result; } @Benchmark public int ifnotElse() { int pos = 0; int result = 0; while (pos < len) { if (pos != 10) { ++pos; } else { ++result; ++pos; } } return result; } @Benchmark public int ifLessthanElse() { int pos = 0; int result = 0; while (pos < len) { if (pos < 10) { ++pos; } else { ++result; ++pos; } } return result; } @Benchmark public int ifGreaterthanElse() { int pos = 0; int result = 0; while (pos < len) { if (pos > 10) { ++pos; } else { ++result; ++pos; } } return result; } @Benchmark public int readMemberVariable_a_byteArray() { int pos = 0; int result = 0; while (pos < len) { result = srcBytes[pos]; pos++; } return result; } }
免责声明:
① 本站未注明“稿件来源”的信息均来自网络整理。其文字、图片和音视频稿件的所属权归原作者所有。本站收集整理出于非商业性的教育和科研之目的,并不意味着本站赞同其观点或证实其内容的真实性。仅作为临时的测试数据,供内部测试之用。本站并未授权任何人以任何方式主动获取本站任何信息。
② 本站未注明“稿件来源”的临时测试数据将在测试完成后最终做删除处理。有问题或投稿请发送至: 邮箱/279061341@qq.com QQ/279061341
软考中级精品资料免费领
历年真题答案解析
备考技巧名师总结
高频考点精准押题
- 资料下载
- 历年真题
193.9 KB下载数265
191.63 KB下载数245
143.91 KB下载数1148
183.71 KB下载数642
644.84 KB下载数2756
相关文章
发现更多好内容- 如何轻松解决 java exe4j 安装问题?(如何解决java exe4j安装问题)
- 如何在 Java 中向 MySQL 数据库添加数据?(java怎么向mysql数据库中添加)
- 如何获取 Java 枚举类的值?(java枚举类的值怎么获取)
- 如何在 Java 中向数据库添加一条数据?(java怎么向数据库添加一条数据)
- 宁夏软考考试科目有哪些?2025年宁夏软考考试科目安排
- Uncomtrade数据库异地备份指南
- CORS 在微服务架构中的应用场景有哪些?(cors在微服务架构中的应用场景)
- 2024下半年陕西软考成绩复查通知
- 如何在 Java 中创建 Maven 项目?(java怎么创建maven项目)
- 2024下半年广西软考成绩复查时间及流程
猜你喜欢
AI推送时光机Java Benchmark 基准测试的实例详解
后端开发2023-05-31
Golang 单元测试和基准测试实例详解
后端开发2024-04-02
gobenchmark基准测试详解
后端开发2023-05-13
Go语言工程实践单元测试基准测试示例详解
后端开发2023-02-05
详解Java使用JMH进行基准性能测试
后端开发2024-04-02
golang函数的性能基准测试详解
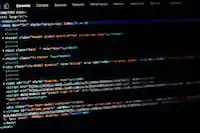
后端开发2024-04-28
Java基准性能测试之JMH的示例分析
后端开发2023-06-20
Sysbench基准测试的示例分析
后端开发2024-04-02
Go语言单元测试和基准测试实例代码分析
后端开发2023-07-05
Java实现手写线程池实例并测试详解
后端开发2023-02-22
Go语言基础单元测试与性能测试示例详解
后端开发2024-04-02
Java基于Rest Assured自动化测试接口详解
后端开发2023-03-21
C语言实现单元测试的示例详解
后端开发2024-04-02
在AmazonAurora中如何实现数据库的性能测试和基准测试
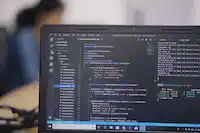
后端开发2024-04-09
在AmazonAurora中如何实现数据库的性能测试和基准测试
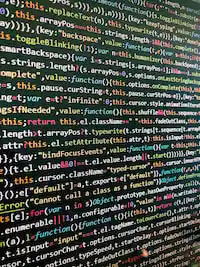
后端开发2024-04-12
基于Python的接口测试框架实例
后端开发2022-06-04
服务器基准测试实践:SysBench的搭建与基本使用
后端开发2023-09-13
咦!没有更多了?去看看其它编程学习网 内容吧